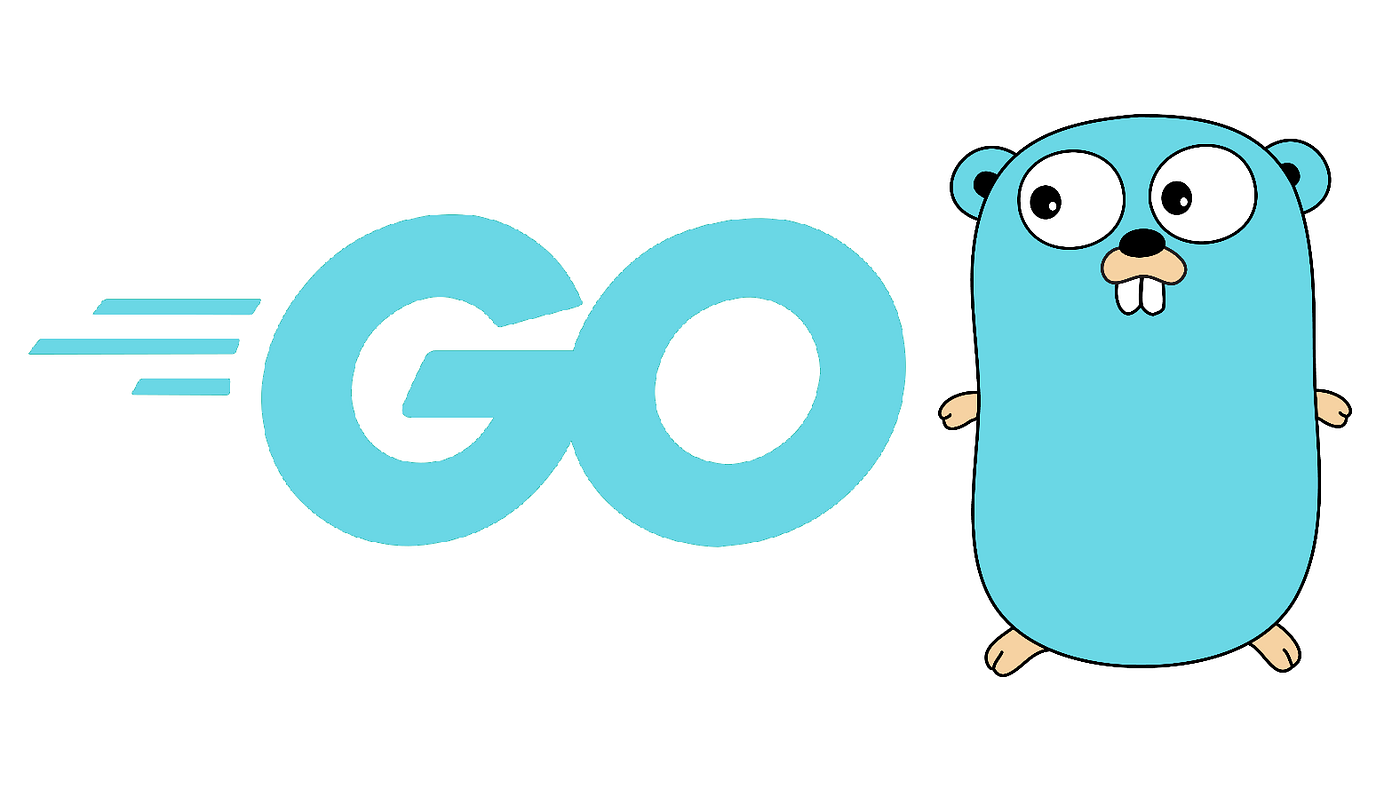
When Slice copies over themselves…..
golang slice copy
This articles clarifies the official slice blog, “Go Slices: usage and internals”.
You can first check this wonderful stackoverflow answer about what the copy function does.
Here I elaborate a little more on the cases when copying with overlapping array. THe official slice blog said:
“The copy function supports copying between slices of different lengths (it will copy only up to the smaller number of elements). In addition, copy can handle source and destination slices that share the same underlying array, handling overlapping slices correctly.”
But wait a minute! What does it mean that “handling overlapping slices correctly”? Here is an example.
package main
import (
"fmt"
)
func main() {
src := []int{10, 11, 12, 13, 14}
dst := src[2:4]
fmt.Println( "Before Copying: dst =", dst)
n := copy(dst, src)
fmt.Println("After Copying: n =", n, "src =", src, "dst =", dst)
}
The results:
Before Copying: dst = [12 13]
After Copying: n = 2 src = [10 11 10 11 14] dst = [10 11]
The destination slice has the length of 2 elements and is smaller than the source slice. So the copy function only copies the first two elements of the source slice to the destination slice.
the destination slice and source slice share the same internal array. The copy function modifies them both. The elements at 0 and 1 positions in the destination slice are exactly by reference the elements at 2 and 3 positions in the source slice.